The evil eye
I’ve been fascinated by the evil eye for many years. Whether intentional or not, I think it’s possible to be negatively affected by someone else’s energy. I like the idea of using a talisman for protection against the evil eye. Talismans vary greatly among the many cultures that believe in the evil eye. I have a necklance and bracelet with evil eye charms and I’ve made talismans out of perler beads. So naturally my next step is to make them in R.
Setting up the coding environment
library(ggplot2)
library(dplyr)
As usual with my pixel art projects, I’m using the ggplot2 package to create the evil eye graphics, and the dplyr package for filtering. Here is a blank theme with a gold background to apply.
eye_theme <- theme(panel.grid = element_blank(),
panel.background = element_rect(fill = "#FFD700"),
axis.text = element_blank(),
axis.ticks = element_blank(),
axis.title = element_blank(),
legend.position = "none")
Symmetry
I like how this type of evil eye symbol is symmetrical with respect to both the x and y axes. I figured I could use that to my advantage when I create the data frame to plot an evil eye. I could essentially figure out one quarter of the pattern and replicate it forward and backwards, and up and down. Here is my evil eye sketch on graph paper. Notice the center point, center column and center row:
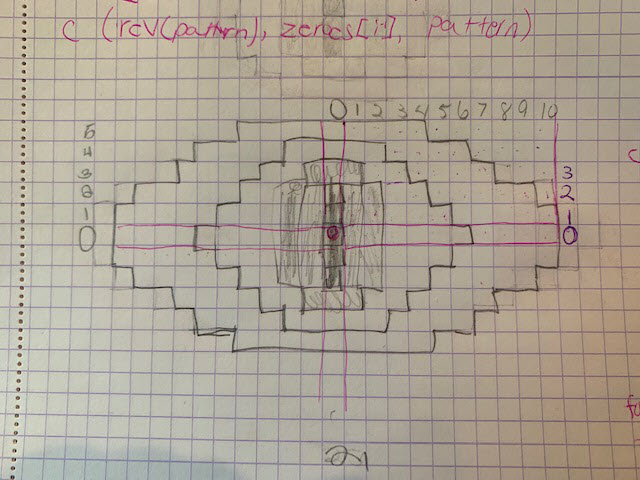
First we’ll create the data frame. We have 3 variables: x
, y
, and c
(for color or fill). The x
and y
values create a grid with a width of 21 (x = -10
to x = 10
) and a height of 11 (y = -5
to y = 5
). The c
variable is initialized to 0 but we will change that when we run the algorithm to assign color values.
#create dataframe 21 x 11
x <- rep(-10:10, each = 11)
y <- rep(-5:5, times = 21)
c <- rep(0, 231)
eye <- data.frame(x, y, c)
I used my pencil sketch as a guide again for the next part: assigning the number of times to repeat each color, starting from y = 0
and moving up to y = 5
. This will work, because each color appears in the same order on every row. The number of times to repeat each color is what changes. Remember I’m only doing this for values where x >= 0
and y >= 0
, because I want to figure out one quarter of the pattern and replicate to the other quadrants. I added a comment here to remember which number is associated with which color.
# dark blue: 1, white: 2, light blue: 3, black: 4
#assign repeat times for each color
black <- c(1, 1, 1, 0, 0, 0)
lt_blue <- c(2, 2, 2, 2, 0, 0)
white <- c(4, 3, 2, 2, 3, 0)
dk_blue <- c(4, 5, 5, 4, 4, 5)
blank <- c(0, 0, 1, 3, 4, 6)
Assigning colors with a loop
This for loop assigns the c
values starting with y = 0
, going up to y = 5
. We create the pattern for each row of y, using the “assign repeat times for each color” that we coded in the previous section. Since I assigned those for cases when x > 0
, we’ll start the reversed version of that pattern (for cases when x <= 0
), followed by a non-reversed version of the pattern. The x = 0
values for c
are covered twice with our pattern, so we can just leave out the first value of the non-reveresed pattern.
# loop to assign c
for(i in 0:5) {
pattern <- c(rep(4, times = black[i+1]),
rep(3, times = lt_blue[i+1]),
rep(2, times = white[i+1]),
rep(1, times = dk_blue[i+1]),
rep(0, times = blank[i+1]))
#assign c for this y
eye$c[y == i] <- c(rev(pattern), pattern[-1])
#assign c for this y, negative version
eye$c[y == -i] <- c(rev(pattern), pattern[-1])
}
After it creates the full pattern for each row of y
, it assigns it to both the positive and negative versions for each y
.
Here is eye #1
This is a common color pattern for evil eye charms.
eye %>%
filter(c > 0) %>%
ggplot(aes(x = x, y = y, fill = factor(c))) +
geom_point(size = 10, shape = 22) +
eye_theme +
scale_fill_manual(values = c("blue", "white", "cyan", "black")) +
xlim(-11, 11) +
ylim(-6, 6)
Let’s make another one
Let’s make one more type of evil eye talisman! It’s the same size so I can reuse my initial x
, y
, and c
values that I assigned already.
#create another dataframe 21 x 11
eye_2 <- data.frame(x, y, c)
Redoing the color patterns:
# white_2: 1, iris_color: 2, black: 3
#assign repeat times for each color
black_2 <- c(1, 1, 1, 0, 0, 0)
iris_color <- c(5, 5, 4, 4, 3, 0)
white_2 <- c(5, 5, 5, 4, 4, 5)
blank_2 <- c(0, 0, 1, 3, 4, 6)
Loop to assign the color values for eye_2
:
# loop to assign c
for(i in 0:5) {
pattern <- c(rep(3, times = black_2[i+1]),
rep(2, times = iris_color[i+1]),
rep(1, times = white_2[i+1]),
rep(0, times = blank_2[i+1]))
#assign c for this y
eye_2$c[y == i] <- c(rev(pattern), pattern[-1])
#assign c for this y, negative version
eye_2$c[y == -i] <- c(rev(pattern), pattern[-1])
}
And now to plot eye_2
, this time with a silver background instead of gold:
eye_2 %>%
filter(c > 0) %>%
ggplot(aes(x = x, y = y, fill = factor(c))) +
geom_point(size = 10, shape = 22) +
eye_theme +
theme(
panel.background = element_rect(fill = "#C0C0C0")
) +
scale_fill_manual(values = c("white", "#00B8D7", "black")) +
xlim(-11, 11) +
ylim(-6, 6)
I like them both. It’s hard to pick a favorite! I hope they’re effective for all of us. ✌️
More evil eyes, this time crocheted
As you can see I’ve been really into evil eyes! I also made some evil eye fingerless gloves, using a pattern by tugboat yarning.